Understanding JavaScript Data Types with Examples: A Guide for Beginner JavaScript Developers.
As we all know, data is the backbone of everything. Data can exist in various forms, and with the help of programming languages, we can perform multiple operations on it. The behavior and structure of these operations may vary depending on the type of data, which leads to different outcomes.
In JavaScript, data is categorized into main two types, primarily based on how it is stored in memory.
Two main Data Types in JavaScript.
- Primitive Data Types
- Non-Primitive Data Types
JavaScript Primitive Data Types
The actual value of the variable will be assigned not the memory reference. Primitive data types can not be changed once created, this behaviour called Immutability.
JavaScript is a dynamically typed language, meaning the type of a variable is determined at runtime by JavaScript, rather than the developer explicitly specifying the data type when declaring the variable in the code.
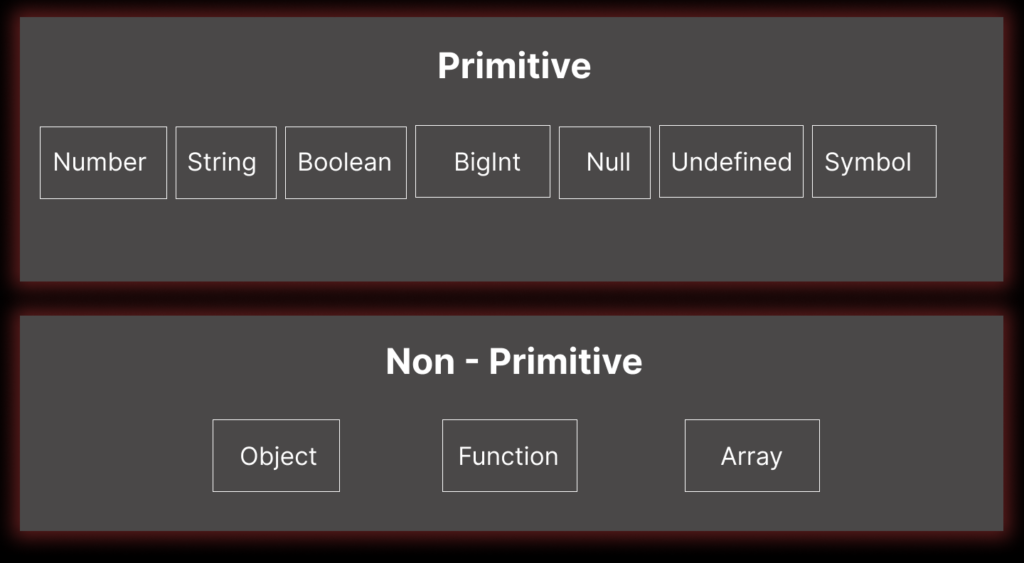
Below mentioned are 7 types of primitive data types.
1. Number
Number data type represents the integer numbers as well as floating-point number.
let num = 10; // variable num stores value 10
let age = 26; // variable age stores value 26
The above statement refers to the declaration of primitive number. Additionally, there is Number Object(non-primitive).
The example of the Number Object as below:
let age = new Number(26); //number 26 stored in number object age
- Programmers prefers to declare the number in primitive way ( let num =10;) unless there is special requirement to declare the number in Object (let num = new Number(10);).
- Primitive numbers are lightweight, easy to use, and perform better, while non-primitive numbers are heavier, harder to use, and can slow down the program.
- NaN – when invalid mathematical operations performed, result will be NaN.
- console.log(“Hello” / 15); // NaN.
2. String
String is the sequance of the characters, the characters can be enclosed in single(‘), double(“) quote or backtick(`).
let firstName = "John"; //String enclosed in double quote
let city = 'London'; //String enclosed in single quote
Javascript provide the option where programmer can insert the expression within string its called Template literals, template literal always enclosed in backticks.
Let age = 25;
Let feedback = `Learning is fun, I am currently ${age+10} years ld`;
The above statements refers to the declaration of primitive value string. Additionally, there is String Object(non-primitive). Declaring a string as an object provides access to multiple advanced string methods. eg. let name = new String(“John”);
Its always suggested to use the primitive type of String instead Object type of String unless there is special requirement.
3. Boolean
Boolean data type stored the logical calue true or false in the variable.
let isActive = true; // Boolean variable hold value true.
- the zero (0) is coerced to false, and non-zero number are coerced to true.
- The empty string coerced to false, and non-empty string coerced to true.
let emptySpace = ''; //Its empty String
console.log(emptySpace == false); // true. Here emptySpace boolean value is false .
let name = 'John'; //not empty string
console.log(name == true); //true. Herename boolean value is true.
let justBornAge = 0;
console.log(justBornAge == false); //true. Here justBornAge boolean value is false.
let updatedAge = 2;
console.log(updatedAge == true);// true . Here updatedAge boolean value is true.Unde
4. Undefined
When a programmer declares a variable without defining it, meaning the variable name is mentioned but no value is assigned, the data type of that variable is undefined.
let age; // variable is onlyn declaired and not yet defined
console.log(age); // Output: undefined
JavaScript is a dynamically typed language, so it determines the variable’s data type at runtime based on the assigned value. Since no value is provided, JavaScript cannot determine a specific type, resulting in the variable having an undefined type.
5. Null
Null is explicitly assigned by the programmer to indicate the absence of a value, and this is done intentionally.
//exmaple 1
let temp = null; // here Temp vaible assigned to the null
//exmaple 2
let person = {
name:"john",
age:12
};
person = null; // cleared the reference of object person
- When we check the data type of null with typeof() then it shows Object is data type of Null.
- Null will always be applied explicitly to variable.
- The most use case of null is to reset the value of variable.
6. BigInt
The BigInt data type was introduced in ES2020 and is used for working with integers beyond the safe range (2^53 – 1). In most cases, if there are no large numbers, the number data type is sufficient instead of BigInt.
let largeNumber = 123456789012345678901234567890n;
console.log(largeNumber + 10n); // Works with BigInt
7. Symbol
Symbol data type instroduced in ES6, its used to create unique and immutable identifiers. Each symbol is the unique, even if two symbol have the same description.
let uniqueId = Symbol("id");
let user = {
name: "Alice",
[uniqueId]: 12345 // Symbol as a unique key
};
console.log(user[uniqueId]); // 12345
- The most use case of symbol is Object property-key.
- Symbol can converted to Boolean and its truthy.
- Symbol can’t be converted into Number
Non-Pimitive Data Types
The memory reference will be assigned to the variable instead the actual value from the memory, its called the non-primitive data types. Object is the non-primitive data type in the javascript and all other complex non-primitive data types falls under the Object data type. Non-primitive datat types are mutable.
1. Object
Object stores the complex data as key-value pairs in block. Whenver we change the value of key then its actually chnage the original value of key from memory. Object dont points to the actual value in memory instead they points to the memory reference of the original value. Object s collection of different different data tyes in key-value pair.
let person ={
name: "Mike",
age: 25
}
How Objects are mutable in JavaScript?
In above code we have created object called person, when we try update the vale of key then actual value of key from memory updates. Please see example below.
person.age = 20;
console.log(person); // Output - {name='Mike', age=20}
If we assign the object to another variable created with “=” operator then only the refernce of the object assigns to the new variable. Hence always clone the Object with Object.assign() or spead operator.
const newPerson = Object.assign({},person); //object.assign() method
const newPerson1 = {...person}; // Spread operator
2. Arrays
Arrays is the collection of the same data types, arrays stores the ordered collection of elements (indexed). Arrays are itterable and accessed with indexs. Below mentioned variable staff holds the memory reference where actually the staff name stored in memory.
let staff =["mike", "Rosie", "Alice"];
3. Function
Object is the block of the code, which executes and returns the unique value. Functions are mutable, below mentioned function addNumber hold the memory address where the block of code are actually present in memory.
function addNumber(a, b)
{
return a + b;
}
Learning and understanding the data types of javascript means creating strong base to learn the language.